Level 1 - Basic Door Creation
Key Objectives
Prerequisites
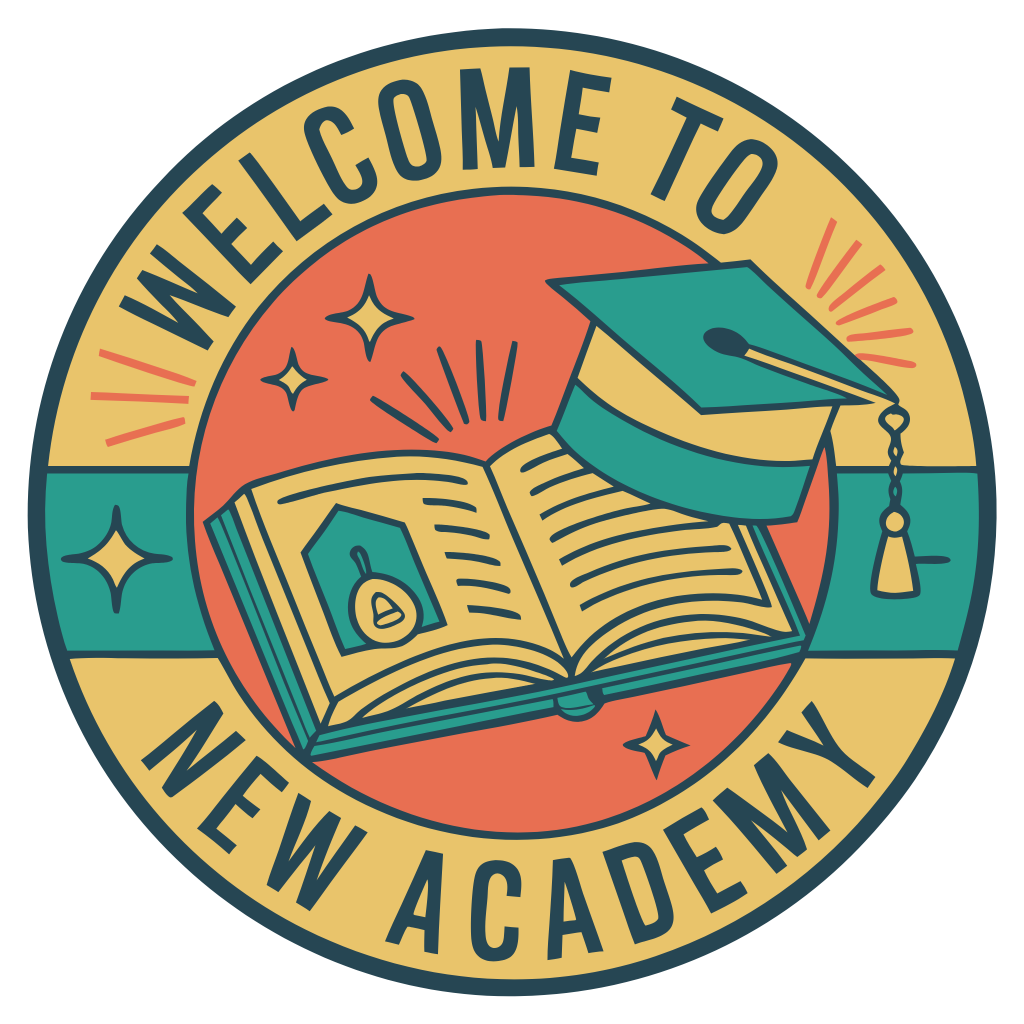
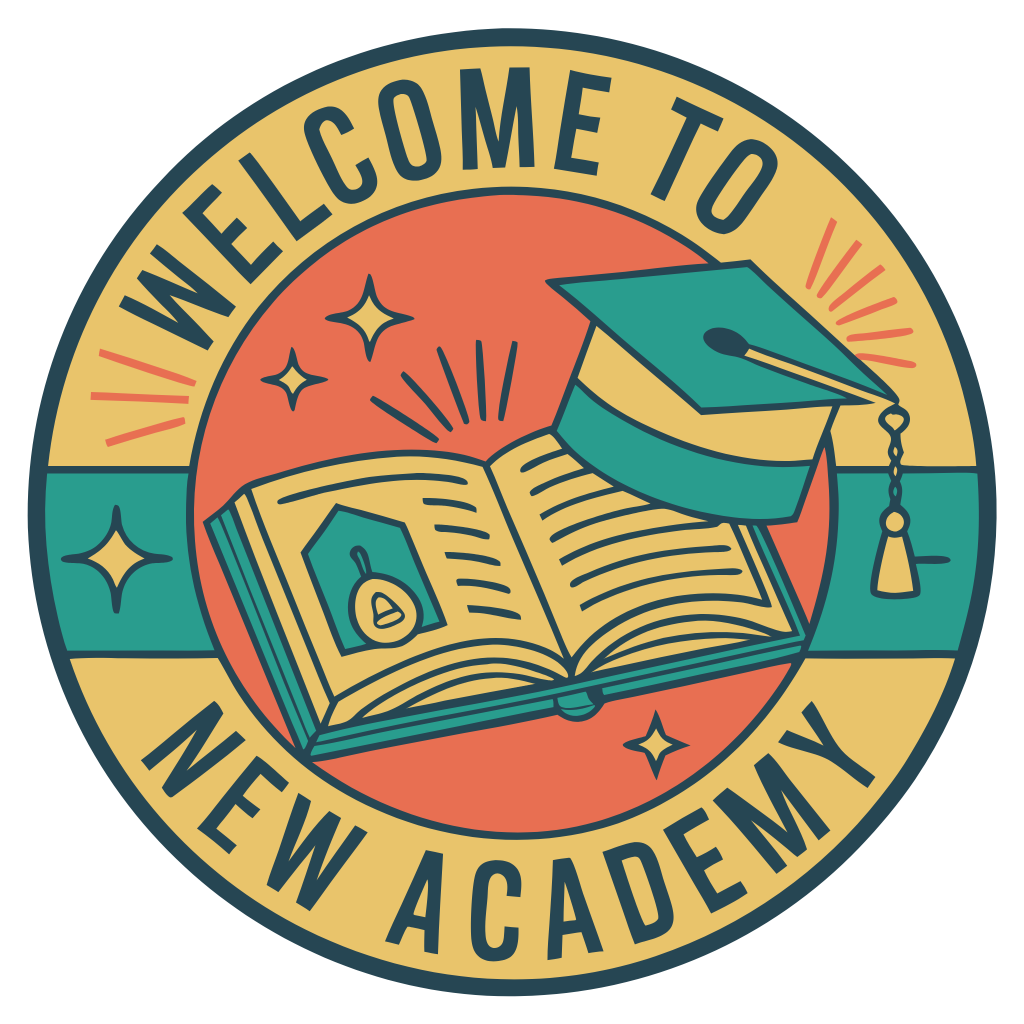
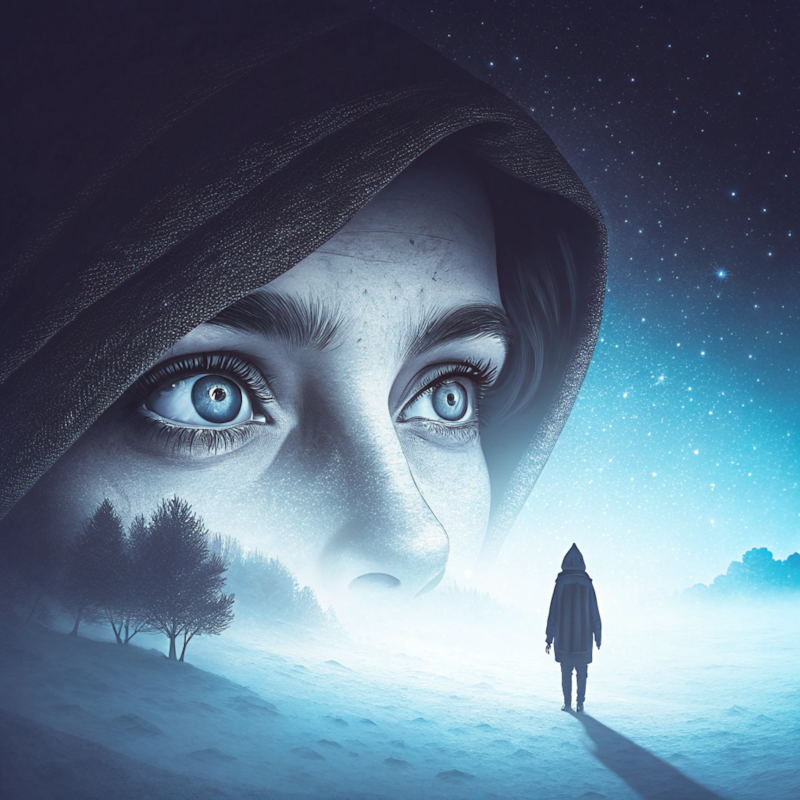
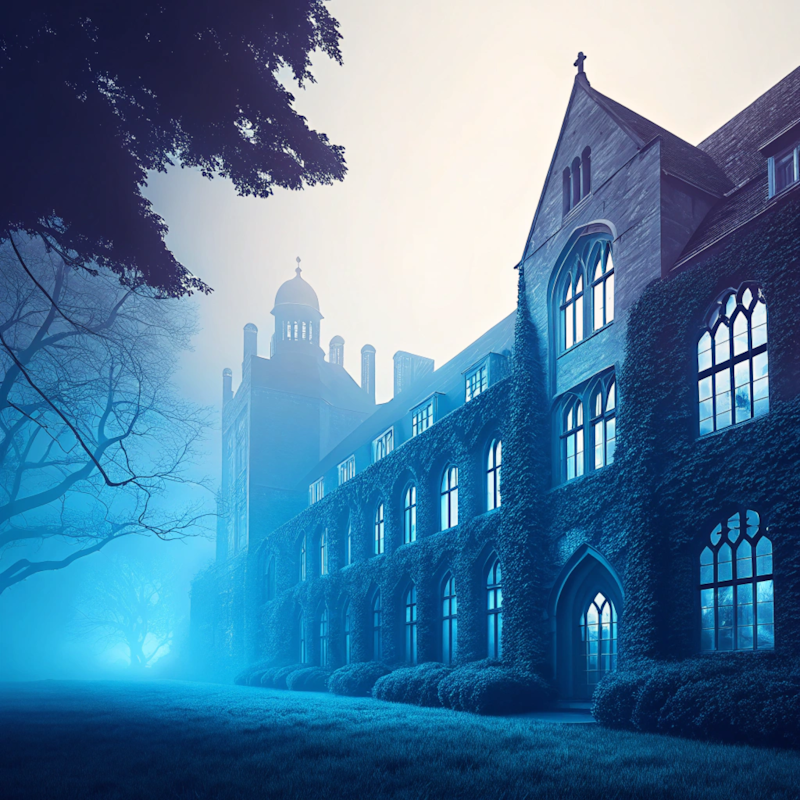
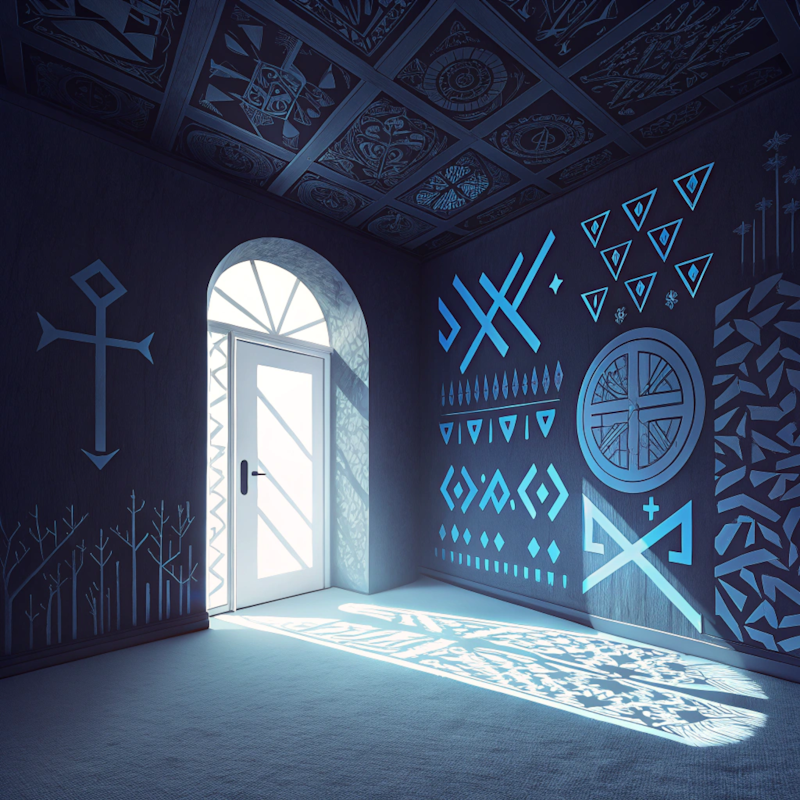
Professor
Let's create an interactive door that not only looks great but sounds great too! We'll use ClickDetector for interaction, TweenService for smooth animations, and add some satisfying sound effects.
Step-by-Step Guide
Step 1: Door Creation
Create Door Frame
- Building the Frame
- Your door frame should look like this
Create Door
- Adding the Door
- Your completed door should look like this
Step 2: Door Scripting
Professor
Now let's make the door interactive with smooth animations and sound effects!
Add Door Script
1local door = script.Parent
2local TweenService = game:GetService("TweenService")
3local isOpen = false
4
5-- Add ClickDetector
6local clickDetector = Instance.new("ClickDetector")
7clickDetector.Parent = door
8clickDetector.MaxActivationDistance = 10
9
10-- Create door sound effects
11local openSound = Instance.new("Sound")
12openSound.SoundId = "rbxassetid://12345" -- Replace with actual sound ID
13openSound.Parent = door
14
15local closeSound = Instance.new("Sound")
16closeSound.SoundId = "rbxassetid://67890" -- Replace with actual sound ID
17closeSound.Parent = door
18
19-- Create animation settings
20local tweenInfo = TweenInfo.new(
21 1, -- Duration
22 Enum.EasingStyle.Quad,
23 Enum.EasingDirection.Out
24)
25
26local function toggleDoor()
27 isOpen = not isOpen
28
29 local targetRotation
30 if isOpen then
31 targetRotation = CFrame.Angles(0, math.rad(90), 0)
32 openSound:Play()
33 else
34 targetRotation = CFrame.Angles(0, math.rad(0), 0)
35 closeSound:Play()
36 end
37
38 local tweenGoal = {
39 CFrame = door.CFrame * targetRotation
40 }
41
42 local tween = TweenService:Create(door, tweenInfo, tweenGoal)
43 tween:Play()
44end
45
46clickDetector.MouseClick:Connect(toggleDoor)
Step 3: Testing
Professor
Time to test our creation! Let's make sure everything works smoothly.
Test Functionality
Congratulations! 🎉



Test your knowledge
Quiz Challenge
Test your knowledge with 2 questions!
What's Next?
Ready for more advanced interactions? Continue to Level 2 - Advanced Interactions where you'll learn to create more complex interactive elements!
Key Concepts Explained
ClickDetector
The ClickDetector is a special object that allows players to interact with parts by clicking on them. It's perfect for interactive elements like doors, buttons, and switches.
TweenService
TweenService is a powerful tool for creating smooth animations. It interpolates (tweens) between different properties over time, creating fluid movements instead of instant changes.
Sound Integration
Adding sound effects provides important feedback to players and makes interactions more satisfying. In this lesson, we've added distinct sounds for opening and closing the door.